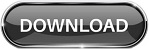

This will give the above indicated, and usually unintended, output. As an illustration, consider this code:ĭef append_to_sequence ( myseq ): myseq += ( 9, 9, 9 ) return myseq tuple1 = ( 1, 2, 3 ) # tuples are immutable list1 = # lists are mutable tuple2 = append_to_sequence ( tuple1 ) list2 = append_to_sequence ( list1 ) print ( 'tuple1 = ', tuple1 ) # outputs (1, 2, 3) print ( 'tuple2 = ', tuple2 ) # outputs (1, 2, 3, 9, 9, 9) print ( 'list1 = ', list1 ) # outputs print ( 'list2 = ', list2 ) # outputs Typically, this behavior of Python causes confusion in functions. Python's built-in id() function, which returns a unique object identifier for a given variable name, can be used to trace what is happening under the hood. This will change s and leave p unaffected, but will change both m and l since both point to the same list object. In our example, assume you set p = s and m = l, then s += 'etc' and l +=. While in most situations, you do not have to know about this different behavior, it is of relevance when several variables are pointing to the same object. However, when used on a mutable object (as in l += ), the object pointed to by the variable will be changed in place. When used on an immutable object (as in a += 1 or in s += 'qwertz'), Python will silently create a new object and make the variable point to it. This becomes tricky, when an operation is not explicitly asking for a change to happen in place, as is the case for the += (increment) operator, for example. As stated above, only mutable objects can be changed in place ( l = 1 is ok in our example, but s = 'a' raises an error). You make the variable point to a different object (newly created ones in our examples). If you assign an object to a variable as below,Ī = 7 s = 'xyz' l = It is important to understand that variables in Python are really just references to objects in memory. Only mutable objects support methods that change the object in place, such as reassignment of a sequence slice, which will work for lists, but raise an error for tuples and strings.
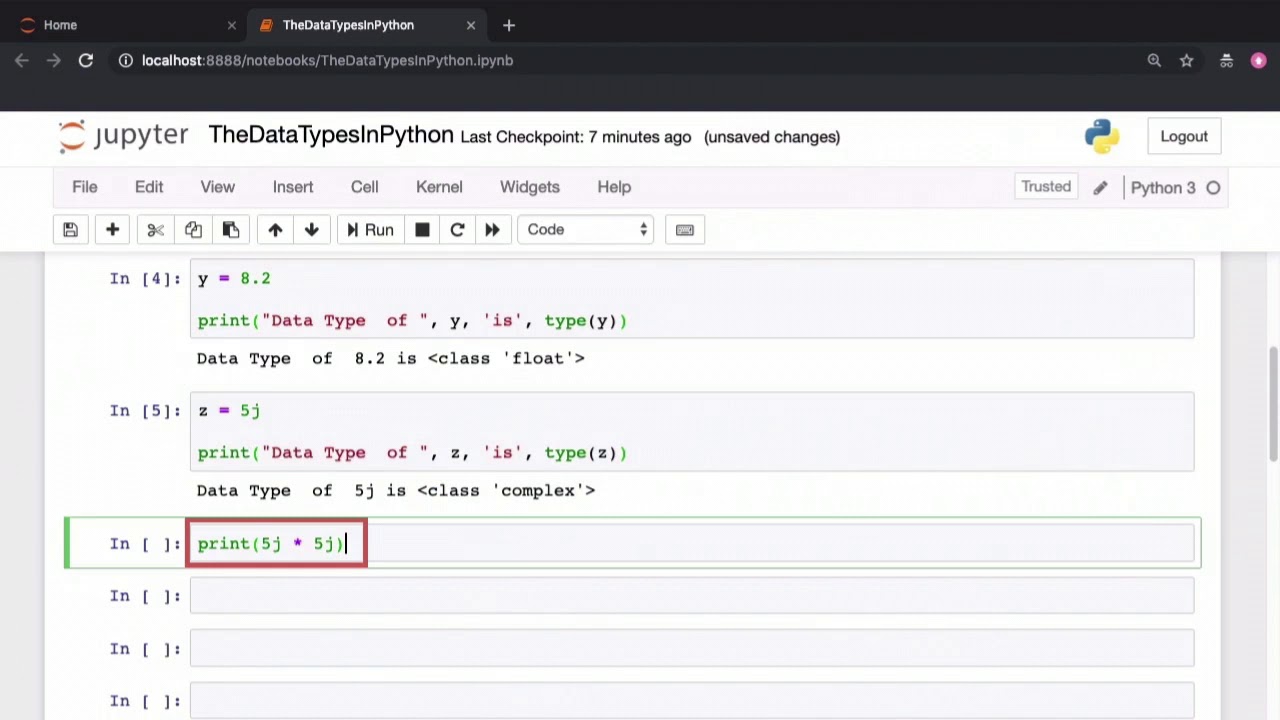
The content of objects of immutable types cannot be changed after they are created. In general, data types in Python can be distinguished based on whether objects of the type are mutable or immutable.
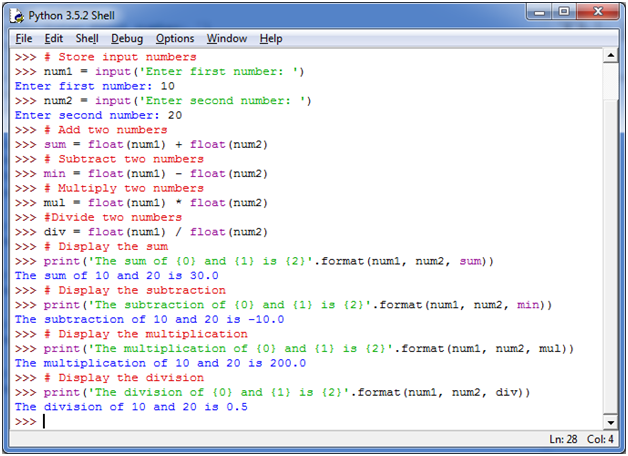
set: an unordered collection of unique objects available as a standard type since Python 2.6.byte array: like bytes, but mutable (see below) only available in Python 3.x.bytes: a sequence of integers in the range of 0-255 only available in Python 3.x.str: String represented as a sequence of 8-bit characters in Python 2.x, but as a sequence of Unicode characters (in the range of U+0000 - U+10FFFF) in Python 3.x.float: Floating-Point numbers, equivalent to C doubles.long: Long integers of non-limited length exists only in Python 2.x.int: Integers equivalent to C longs in Python 2.x, non-limited length in Python 3.x.In fact, conditional expressions will accept values of any type, treating special ones like boolean False, integer 0 and the empty string "" as equivalent to False, and all other values as equivalent to True. Mostly interchangeable with the integers 1 and 0. Useful in conditional expressions, and anywhere else you want to represent the truth or falsity of some condition. boolean: the type of the built-in values True and False.Some of the types are only available in certain versions of the language as noted below. Sticking to the hierarchy scheme used in the official Python documentation these are numeric types, sequences, sets and mappings (and a few more not discussed further here). Python's built-in (or standard) data types can be grouped into several classes.
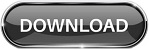